Make image background transparent with CSS
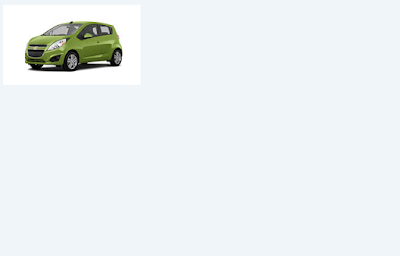
If you have a opaque image and it doesn't align with the background of your webpage, you can make the background transparent with CSS < style > .bg-blend{ background: url("https://images.hertz.com/vehicles/220x128/ZEUSECAR999.jpg"); background-color: #f0f5f9; background-blend-mode: multiply; height: 128px; width: 220px; } </ style > < body > < div class =" bg-blend "></ div > </ body >